In Linux, each process has their own independent memory spaces. For any specific process, its memory cannot be accessed by the other processes. The shared memory allows different processes visit the same memory space, which is the most efficient way to share and pass data.
There are four operations for shared memory:
shmget
function acquires or creates shared memoriesshmat
function connects the shared memory to the current processshmdt
separates the shared memory from the current processshmctl
deletes the shared memory. Usually when the shared memory is created, it doesn’t get deleted unless the
whole program terminates.
Create a shared memory
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/shm.h>
struct st_pid
{
int pid;
char name[51];
};
int main(int argc, char* argv[])
{
int shmid;
if((shmid = shmget(0x5005, sizeof(struct st_pid), 0640|IPC_CREAT))==-1)
{
printf("shmget(0x5005) failed\n");
return -1;
}
return 0;
}
Compile, run and check shared memory with ipcs -m
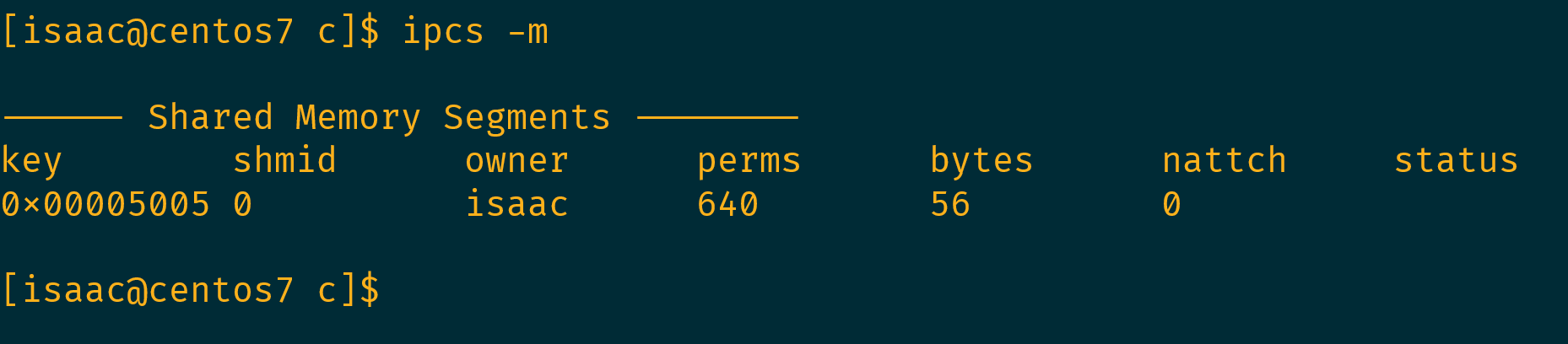
We can delete this shared memory with ipcrm -m shmid
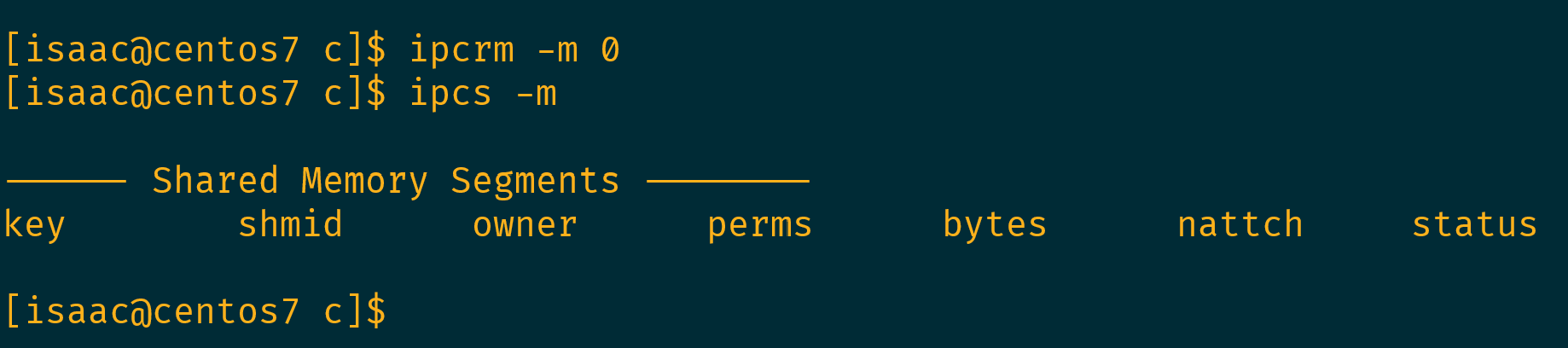
Other operations
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/shm.h>
struct st_pid
{
int pid;
char name[51];
};
int main(int argc, char* argv[])
{
// assign a variable for the shared memory
int shmid;
// acquire or create a shared memory, key is 0x5005
if((shmid = shmget(0x5005, sizeof(struct st_pid), 0640|IPC_CREAT))==-1)
{
printf("shmget(0x5005) failed\n");
return -1;
}
// used for the struct variable pointing to the shared memory
struct st_pid *stpid=0;
// connect the shared memory to the current process
if((stpid=(struct st_pid *)shmat(shmid, 0, 0))==(void *)-1)
{printf("shmat failed\n"); return -1;}
// check before assigning
printf("pid=%d, name=%s\n", stpid->pid, stpid->name);
stpid -> pid = getpid();
strcpy(stpid -> name, argv[1]);
// check after assigning
printf("pid=%d, name=%s\n", stpid->pid, stpid->name);
//separate the shared memory from the current process
shmdt(stpid);
return 0;
}
Compile and run
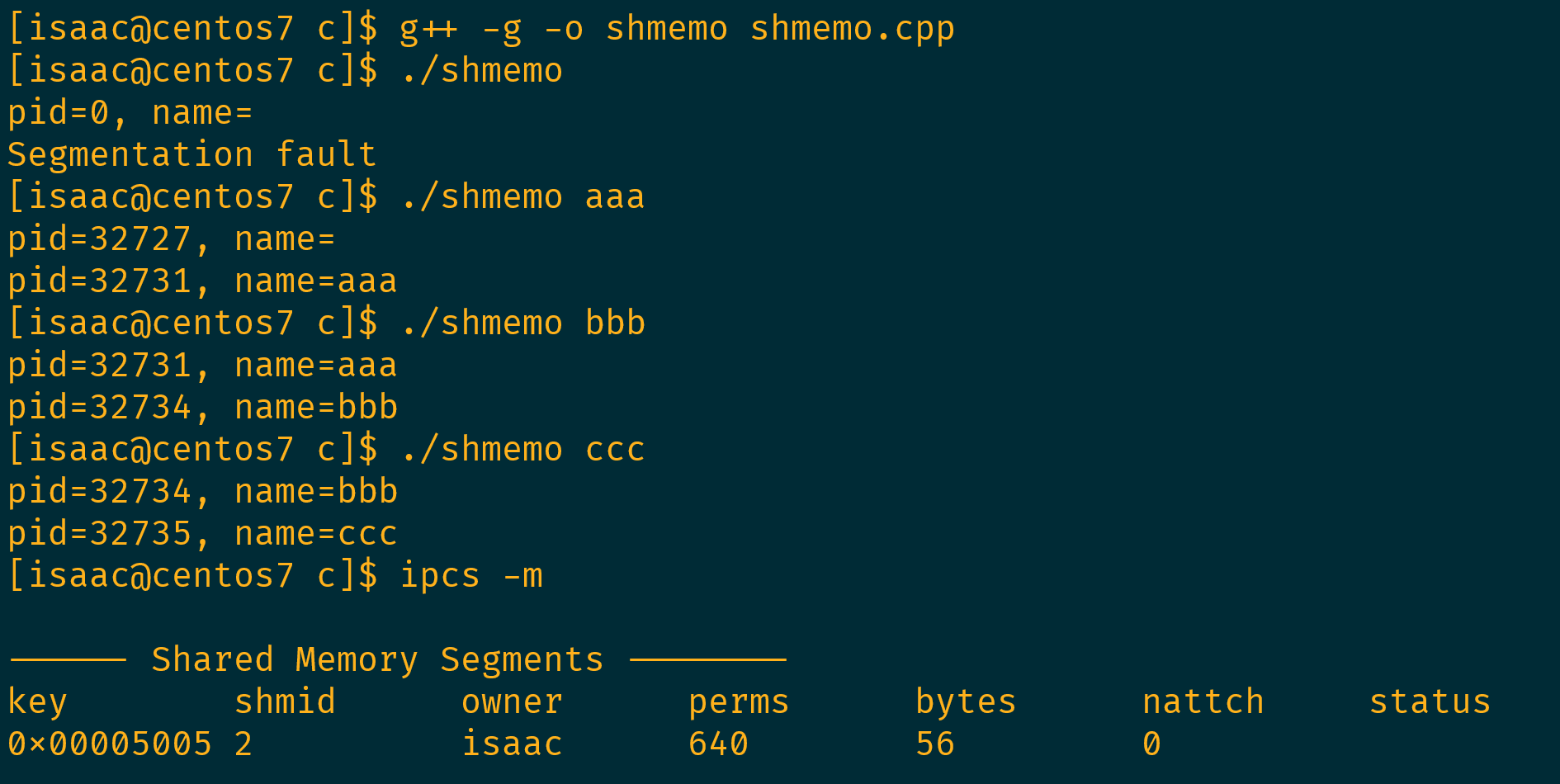
Delete the shared memory
We can use shmctl
to delete the shared memory. This function can actually perform more than just deletion.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/shm.h>
struct st_pid
{
int pid;
char name[51];
};
int main(int argc, char* argv[])
{
// assign a variable for the shared memory
int shmid;
// acquire or create a shared memory, key is 0x5005
if((shmid = shmget(0x5005, sizeof(struct st_pid), 0640|IPC_CREAT))==-1)
{
printf("shmget(0x5005) failed\n");
return -1;
}
// used for the struct variable pointing to the shared memory
struct st_pid *stpid=0;
// connect the shared memory to the current process
if((stpid=(struct st_pid *)shmat(shmid, 0, 0))==(void *)-1)
{printf("shmat failed\n"); return -1;}
// check before assigning
printf("pid=%d, name=%s\n", stpid->pid, stpid->name);
stpid -> pid = getpid();
strcpy(stpid -> name, argv[1]);
// check after assigning
printf("pid=%d, name=%s\n", stpid->pid, stpid->name);
//separate the shared memory from the current process
shmdt(stpid);
//delete the shared memory
if(shmctl(shmid, IPC_RMID, 0) == -1)
{
printf("shmctl failed\n"); return -1;
}
return 0;
}
Compile, run and check the shared memory.
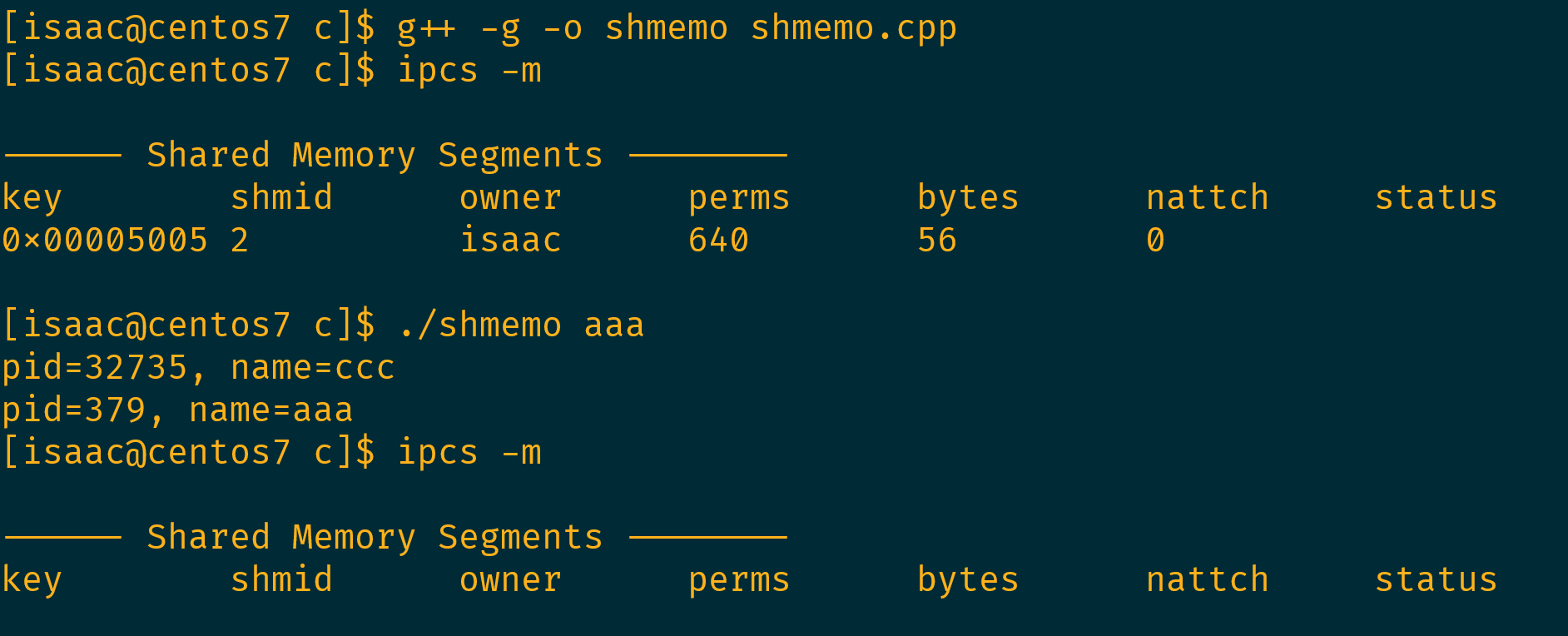
The memory was deleted.