Python has offered a number of built-in data structures e.g. list
, dict
, set
etc. Each
of them has already implemented very useful methods and attributes that can be applied to
construct more complex data structures.
Object Oriented vs. Process Oriented
For beginners, it’s very convenient to use Python in a more “Process Oriented”
manner, e.g. write a script to parse some data, to clean some files etc.
However, for “Objected Oriented Programming”, the process is quite different.
OOP abstracts the real-world “objects” into something called “classes”. Classes
usually include attributes and methods to better describe those “objects”.
Everything is an object in Python.
In Python, class
is used to define a class. We can substantiate the associated objects by
invoking the class’ constructor function. So an object is an instance of a class, whereas a
class is an abstraction of an object.
Three Key Features
There are three most commonly known features for OOP: Encapsulation, Inheritance and
Polymorphism.
Encapsulation
Encapsulation means making some API public while hiding implementation details. e.g. forlist
, most people probably don’t know how it’s built in Python, yet we can easily call its
methods such as .append()
, .pop()
etc.
Inheritance
Inheritance means reusing attributes and methods of the parent class.
Polymorphism
Polymorphism means for different objects, if they invoke the same class, they may generate
different results or have different explanations.
Here’s an example:
class Animal:
def __init__(self, name, age):
self.name = name
self.age = age
def set_name(self, new_name):
self.name = new_name
def speak(self):
print(f"My name is {self.name}")
def main():
a = Animal("Lasse", 10)
a.speak()
if __name__=="__main__":
main()
Run the script and here’s the output
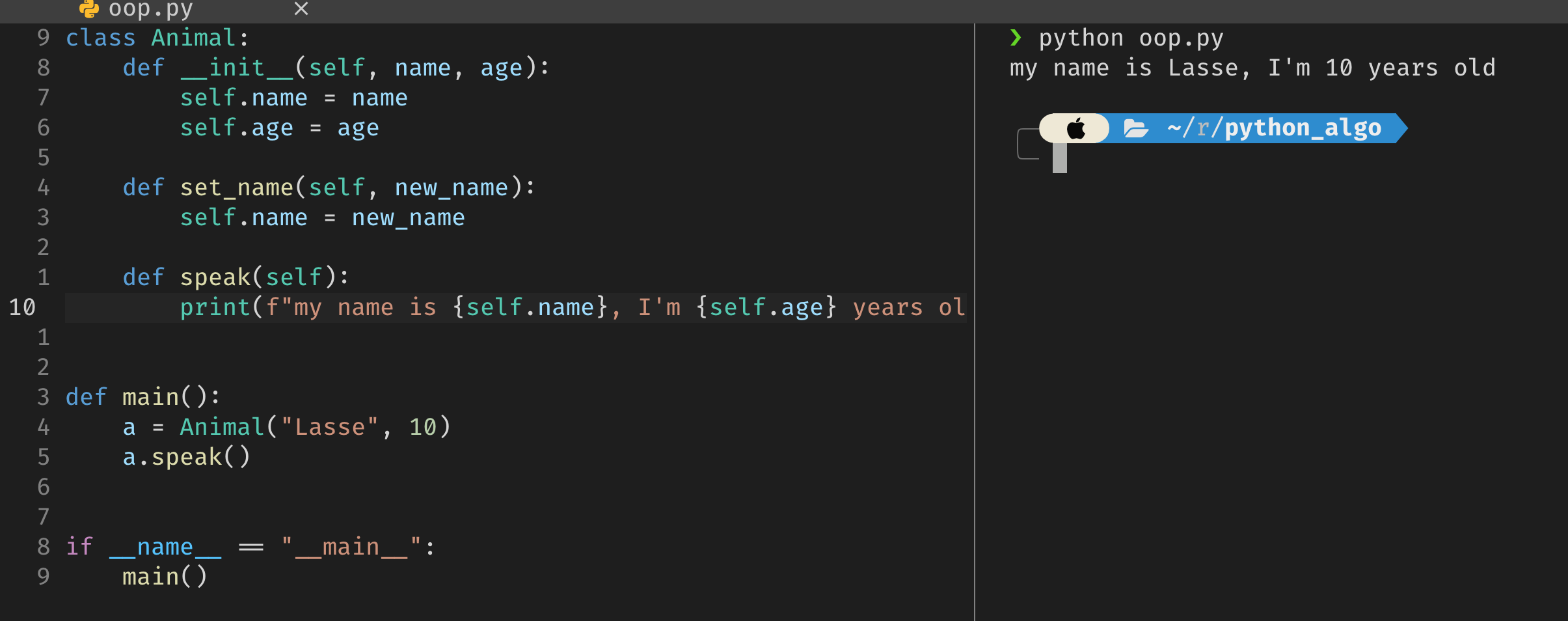
We add a child class Pig
to inherit from the parent class Animal
class Animal:
def __init__(self, name, age):
self.name = name
self.age = age
def set_name(self, new_name):
self.name = new_name
def speak(self):
print(f"my name is {self.name}, I'm {self.age} years old")
class Pig(Animal):
def sleep(self):
print(f"{self.name} is sleeping")
def main():
a = Animal("Lasse", 10)
a.speak()
p = Pig("Peggy", 2)
p.speak()
p.sleep()
if __name__ == "__main__":
main()
If we run the above script, we will get the following output:
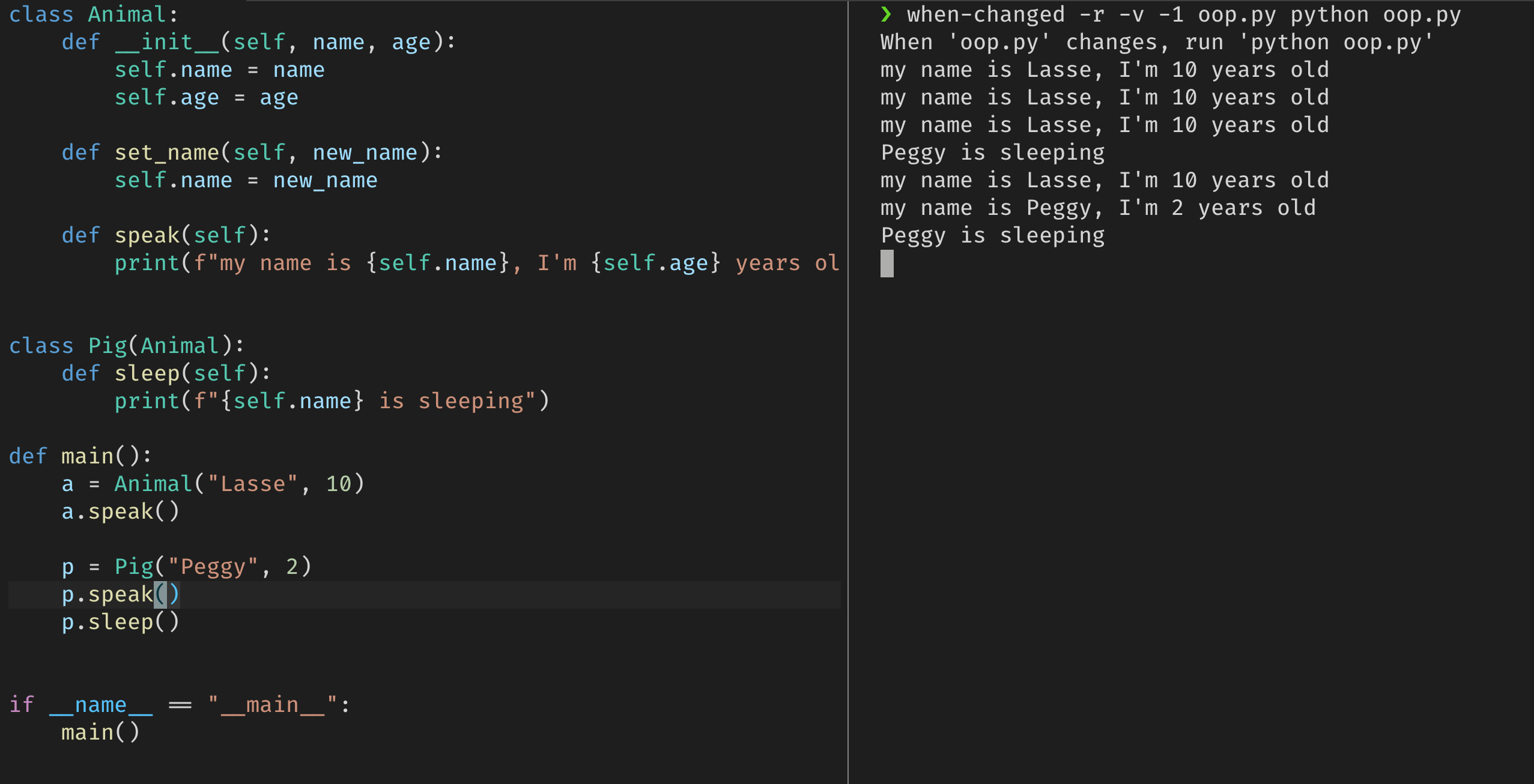
Class Pig has inherited all the attributes and methods from the parent class Animal.
For Polymorphism, we add another class Cat but assign different behavior to the speak
method:
class Animal:
def __init__(self, name, age):
self.name = name
self.age = age
def set_name(self, new_name):
self.name = new_name
def speak(self):
print(f"my name is {self.name}, I'm {self.age} years old")
class Pig(Animal):
def sleep(self):
print(f"{self.name} is sleeping")
class Cat(Animal):
def speak(self):
print(f"meow...my name is {self.name}...meow...,I'm {self.age} years old")
def main():
a = Animal("Lasse", 10)
a.speak()
p = Pig("Peggy", 2)
p.speak()
p.sleep()
c = Cat("Garfield", 3)
c.speak()
if __name__ == "__main__":
main()
Run the above script, we can see for the same method, class Dog and Cat may have different
results:
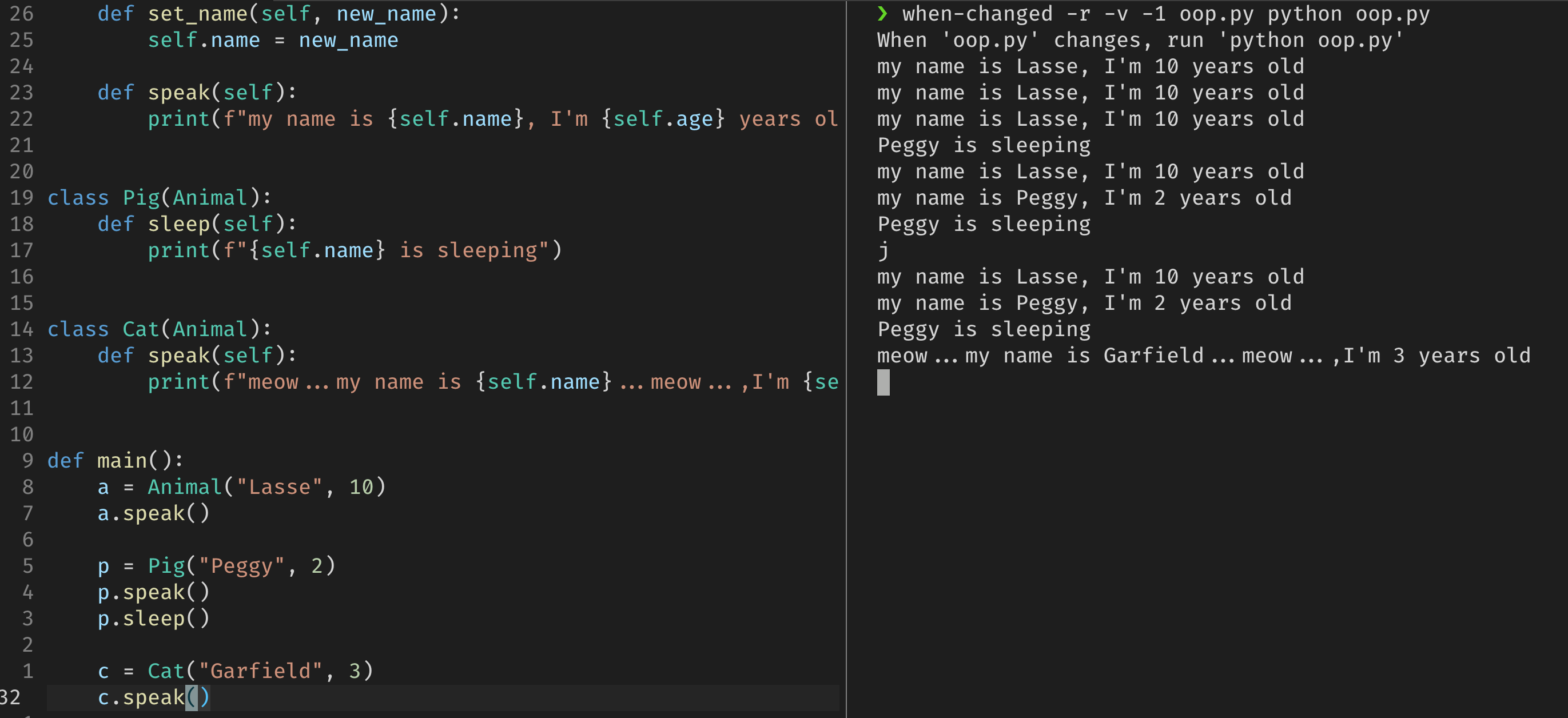